
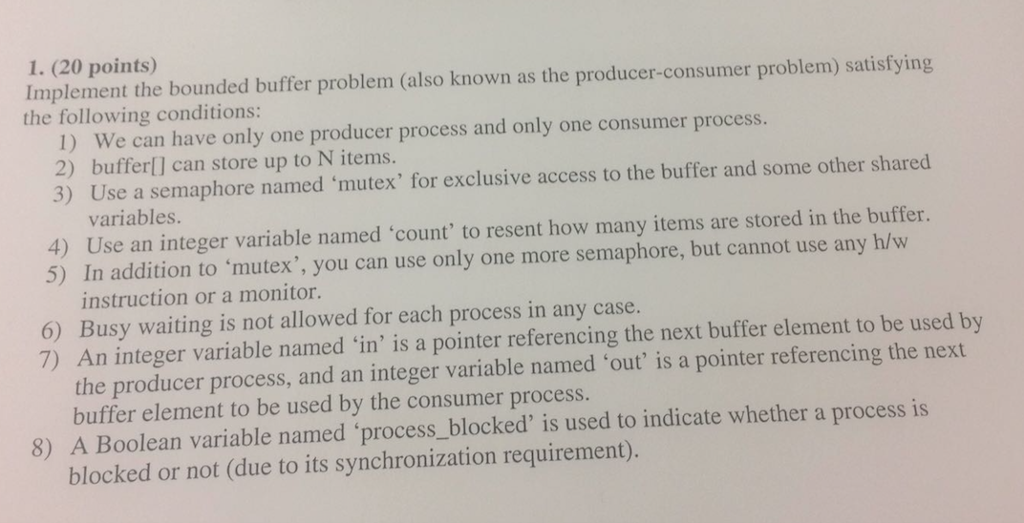
- #Producer consumer with bounded buffer binary semaphor how to#
- #Producer consumer with bounded buffer binary semaphor full#
Each semaphore maintains a FIFO (first-in-first-out) queue of blocked processes. There are multiple producer processes, referred to as Pa, Pb, Pc, etc., and multiple consumer processes, referred to as Ca, Cb, Cc, etc.
#Producer consumer with bounded buffer binary semaphor full#
The mutex semaphore makes sure there are no 2 processes working in the buffer at the same time, so I don't see how this property can possibly change if you have more processes. Semaphores empty and full are linear semaphores that can take unbounded negative and positive values. Why will this not work if I have more than 1 producer and/or more than 1 consumer? I've looked everywhere, but I can't find an answer I understand :s. The typical approach for 1 consumer and 1 producer looks like this : Producer : It appears in my lecture notes, but I simply cannot understand why the single consumer/producer approach won't work.
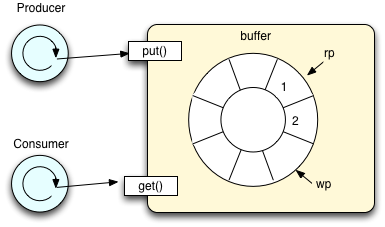
Moi python $ python boundedbuffer_semaphore.I'm a bit stuck on the multiple consumer/producer problem. Print("'".format(buf, buf, consumer_idx)) Dijkstra found the solution for the producer-consumer problem as he worked as a consultant for the Electrologica X1 and X8 computers: 'The first use of producer-consumer was partly software, partly hardware: The component taking care of the. Producer_idx = (producer_idx + 1) % buf_size In computing, the producer-consumer problem (also known as the bounded-buffer problem) is a family of problems described by Edsger W. Global producer_idx, counter, buf, buf_size
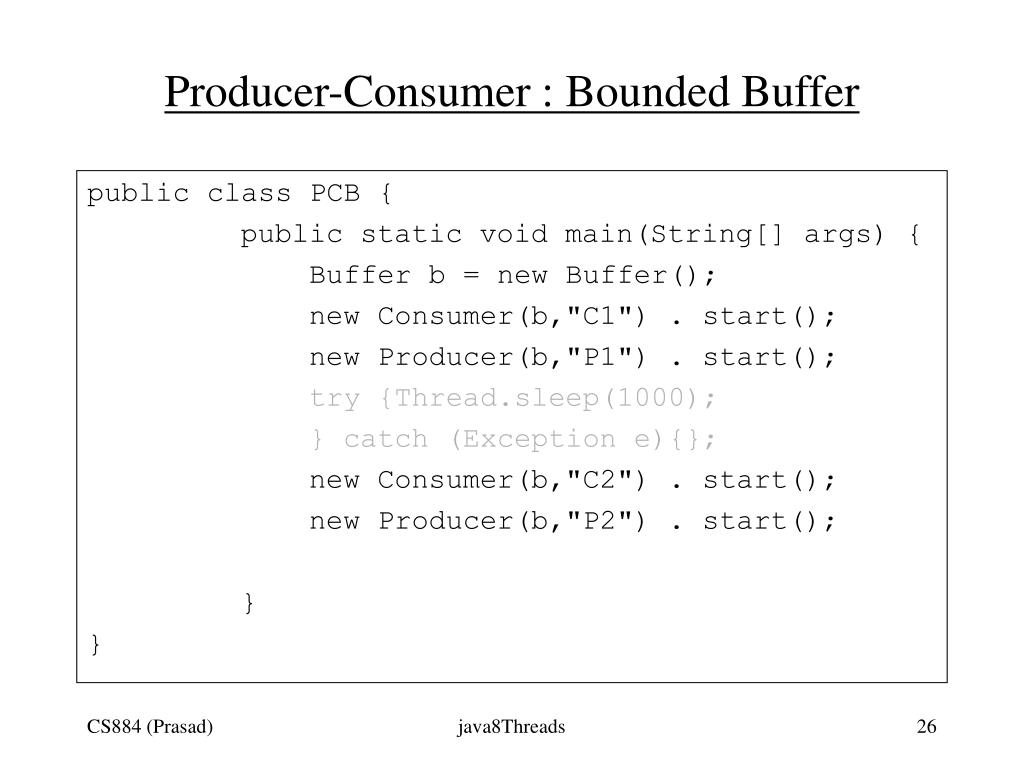
Program Because we have two types of threads, the producer and the consumer, we have two classes ProducerThread and ConsumerThread. Raise ValueError('Result size is %d instead of %d' % (len(result), value_count)) The binary semaphore for locking the buffer should have an initial value 1, which is obvious. Here's a small test program I used to play around: def producer(queue, start, end, step):ĭef consumer(queue, count, result, lock): Self.read_index = (self.read_index + 1) % len(self.buff) Self.write_index = (self.write_index + 1) % len(self.buff) The same memory buffer is shared by both producers and consumers which is of fixed-size. Also, I think you could access the buffer without holding the locks (because lists themselves are thread-safe): def put(self, val): I would remove the size attribute in favor of len(self.buff) though and rename the start and end indices to read_index and write_index respectively (and the locks as well). ( CPython's queue implementation does this.) You could remove one of the locks and use it in both get and put to protect both the start and the end index which wouldn't allow consumers and producers to access the queue simultaneously.
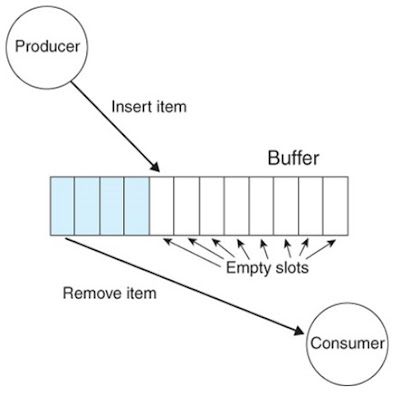
The two semaphores are definitely necessary. The producer and consumer processes are identical one can think of the producer as. The semaphores prevent concurrent producers and consumers from writing and reading too much and the locks prevent concurrent producers or consumers from modifying the end or start indices simultaneously. Mutex) controls access to the Critical Section. Is this implementation bug-free? Could this be simplified further to use fewer mutexes/semaphores? The consumer will also sleep for a random. Random numbers will be produced using the rand() function, which produces random integers between 0 and RANDMAX. The producer and consumerrunning as separate threadswill move items to and from a buffer that is synchronized with these empty, full, and mutex structures. Self.closed = Semaphore(size) # block till there's item to consumeįor _ in range(size): # initialize with all closed acquired so that consumer is blocked Producer and Consumer Threads Producer and Consumer Threads The producer thread will alternate between sleeping for a random period of time and inserting a random integer into the buffer. Self.open = Semaphore(size) # block till there's space to produce Self.start_lock = Lock() # protect start from race across multiple consumers Self.end_lock = Lock() # protect end from race across multiple producers
#Producer consumer with bounded buffer binary semaphor how to#
I'm trying to understand how to implement a Queue with a bounded buffer size that can be used by multiple producers and consumers using Python Semaphores.
